Exponents are a fundamental mathematical concept that is frequently used in computer programming. In Java, exponents can be calculated using built-in functions and mathematical operators. This article provides a comprehensive overview of what exponents are and how to perform exponent calculations in Java.
What is Exponent & How to Do Exponents in Java? Unfortunately, like some other widely used computer languages, Java does not come with an exponent operator, which means you have to do exponents through another method. Alternatively, in Java, the common math operations can be suitably titled Java .util.Math in the math static class.
This will support the following operation: common trigonometry, absolute value, exponents, and rounding. It is most common for the results of the mathematical operations to be “double” data types, which can be cast down to floats and integers if required. As well as you can take help from any offshore JavaScript development to get this task done. But if you already decided to DIY, then….
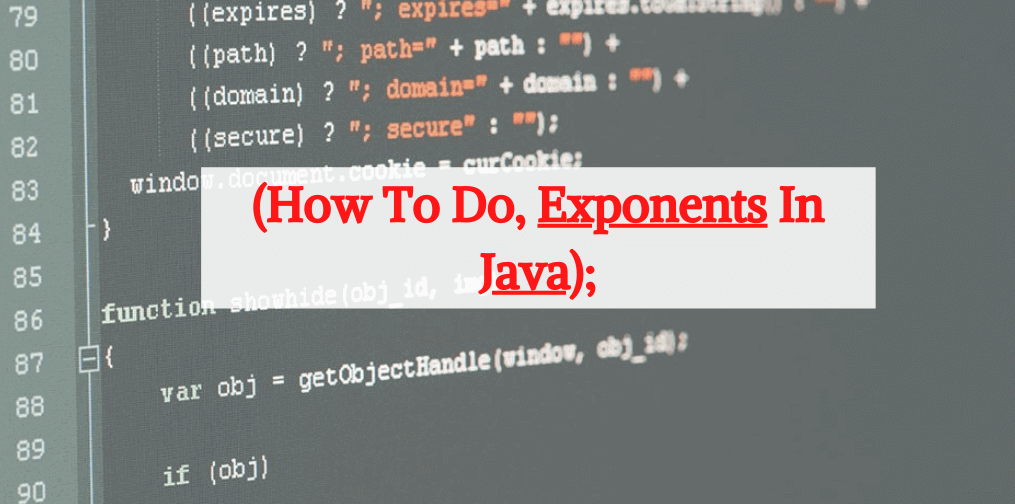
Table of Contents
What are Exponents?
Exponents are a mathematical concept used to represent repeated multiplication. For example, 2 raised to the power of 3 (written as 2^3) means 2 multiplied by itself 3 times, resulting in 8. Exponents are denoted using a base number and a superscript exponent, indicating the number of times the base is multiplied by itself.
Exponents are useful in computer programming for performing complex calculations and simplifying code. Java provides built-in functions for exponent calculations, making it easy to include exponents in your code.
Exponents in Java
There are several ways to perform exponent calculations in Java, including using the Math.pow() function and the exponent operator (**). The Math.pow() function is a built-in Java function that takes two arguments: the base number and the exponent. The function returns the value of the base raised to the power of the exponent.
Using Math.pow() for Exponent Calculation
To use Math.pow() in your Java code, you must first import the Math class. The syntax for using Math.pow() is as follows:
import java.lang.Math;
double result = Math.pow(base, exponent);
How to Do Exponents in Java
Following are the steps for doing exponent in Java:
- Start with opening the Java editor you prefer, such as the Netbeans Integrated Development Environment, or the IDE.
- The create a new source file by selecting “File” and “New Class” or open an existing Java source file.
- At the very top of the document, for Java exponent type the following line:
import java.util.Math; - Then, anywhere in the document, input the following formula to find the exponent Java:
double result = Math.pow(number, exponent);
Swap “number” with the base value and the value of the “exponent.”
The Math.pow() function takes two arguments: the base number (as a double) and the exponent (as a double). The function returns the value of the base raised to the power of the exponent. The result of the calculation is then stored in the variable “result”, which is also a double.
Example Code for Exponent Calculation
Here is an example code snippet that demonstrates how to use Math.pow() for exponent calculation in Java:
import java.lang.Math;
public class ExponentCalculation {
public static void main(String[] args) {
double base = 2;
double exponent = 3;
double result = Math.pow(base, exponent);
System.out.println(base + ” raised to the power of ” + exponent + ” is ” + result);
}
}
When you run this code, the output will be:
2.0 raised to the power of 3.0 is 8.0
For example:
double result = Math.pow(10,5);
You will get the result: 100,000, or 10^5.
Additionally, there are some special considerations to be mindful of when to Pow () method is used for exponent in Java; they are as follows:
- In case the second value is zero, whether positive or negative, the result will be 1.0.
- In case the second value is one, then the resulting value would be the same as the first number.
- In case the second value is N, then the output will also be N.
For Loop Method
If the number you are raising is 1 or greater, you can also try the loop method. Although the technique is lengthy, thankfully, you can skip some work using the existing code provided below to copy and paste directly to Java IDE to skip writing the code entirely by yourself.
You can use the code given below by replacing the numbers with your own values that you need to calculate and then run the code:
package exponent_example;
public class Exponent_example {
public static void main(String[] args) {
double num = 2;
int exp = 3;
double answer = Pow(num, exp);
System.out.println(answer);
}
public static double Pow(double num, int exp){
double result =1;
for (int i = 0; i < exp; i++) {
result *= num;
}
return result;
}}
Replace ‘num = 2’ with the base number and ‘exp = 3’ with the power. It is important that the exponent value is an integer, which is denoted by the “int.” The necessity of the integer is one of the limitations of this method.
Tips for Using Exponents in Java
Here are some tips to keep in mind when using exponents in Java:
- Remember to import the Math class before using Math.pow().
- Be sure to specify the base and exponent as doubles when using Math.pow().
- Use the exponent operator (**), if it is more convenient in your code.
- Remember that the result of an exponent calculation is a double, so be sure to store the result in a double variable.
FAQs
There is no operator, but there is a method. Math.pow(2, 3) // 8.0 Math.pow(3, 2) // 9.0. FYI, a common mistake is to assume 2 ^ 3 is 2 to the 3rd power. It is not. The caret is a valid operator in Java (and similar languages), but it is binary xor.
exp() is used to return the Euler’s number e raised to the power of a double value. Here, e is an Euler’s number and it is approximately equal to 2.718281828459045.
Squaring a number in Java can be accomplished in two ways. One is by multiplying the number by itself. The other is by using the Math. pow() function, which takes two parameters: the number being modified and the power by which you’re raising it.
Conclusion
Exponents are a powerful mathematical concept that is frequently used in computer programming. Java provides built-in functions and operators for performing exponent calculations, making it easy to include exponents in your code. By using the Math.pow() function and following a few tips, you can easily perform exponent calculations in Java. So, start practicing and incorporating exponents in your Java programs to take them to the next level.